- Published on
Mixins and Composition in Typescript Part 3
- Authors
- Name
- Martin Karsten
- @mkdevX
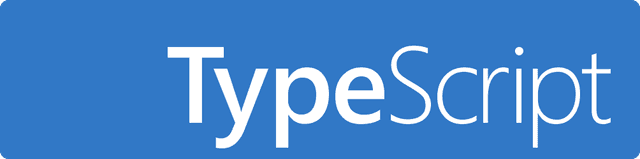
Let's look out how to use higher order functions to achieve reusablity. In Part 1 we learned how to use Mixins to achieve code reusablity. In Part 2 we used composition to achieve reusablity. In Part 3 we will use higher order functions to achieve reusablity.
Higher order Functions
We define two interfaces
interface CanFly {
fly(): void
}
interface CanSwim {
swim(): void
}
Next we implement two higher order functions. In the previous parts we used interfaces instead
function fly() {
console.log('I can fly!')
}
function swim() {
console.log('I can swim!')
}
Now, we create a higher-order function that takes an object and a set of methods to be added to that object:
function withMethods<T extends object, M extends object>(base: T, methods: M): T & M {
return Object.assign(base, methods)
}
Laslty we create a Duck class that will call fly
and swim
class Duck {}
const duck = withMethods(new Duck(), { fly, swim })
duck.fly() // Output: I can fly!
duck.swim() // Output: I can swim!
Conclusion
Reusability is achievable with different approaches. Mixins, Composition or higher order functions are just a few possibilities.